0.概述
string是C++标准库的一个重要部分,本意是字符串,和字符数组不同的是,字符数组是通过一个一个字符模拟的字符串,而string本身就是字符串,string在处理字符串问题时,十分强大。
1.字符和字符串的区别
在C++语言中,字符和字符串有着严格的区别,
char c; // 字符, c=‘s’; // 字符的赋值用单引号
char ary[10]; //字符数组 cin>>ary; //字符数组的赋值一般用cin或scanf("%s",ary),但是这两种方法都不能读入包含空格的字符串
string s; //字符串 s="hello world"; //字符串的赋值用双引号
3.字符串的输入和输出
使用string,建议引入string 头文件
#include<iostream> #include<string> #include<cstdio> using namespace std; int main() { string s; // 定义一个字符串 getline(cin,s); //输入一个字符串 cout<<s; //输出字符串 return 0; }
4.字符串的遍历
要去遍历一个字符串,就要知道字符串的长度,在C++中,可以通过
字符串名.size 和 字符串名.length 来确定字符串的长度
#include<iostream> #include<string> #include<cstdio> using namespace std; int main() { string s; // 定义一个字符串 getline(cin,s); // 输入一个字符串 cout<<"字符串的长度:"<<s.size()<<endl; cout<<"字符串的长度:"<<s.length()<<endl; return 0; }
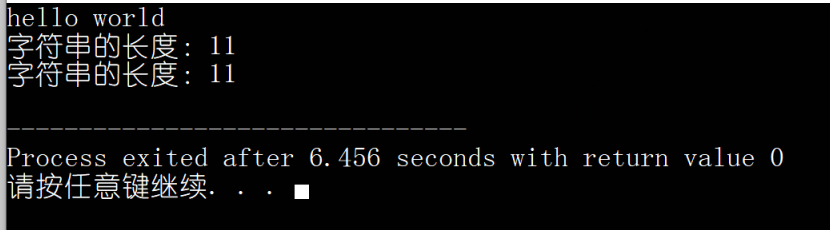
可以看出,两者作用类似。而且输出的字符串的长度是包含字符单词和单词之间的。
知道了一个字符串的长度,就可以通过for循环去遍历字符串了,比如下面的这个例子:
输入一段字符,查找其中数字的个数
#include<iostream> #include<string> #include<cstdio> using namespace std; int main() { string s; // 定义一个字符串 int sum=0; getline(cin,s); // 输入一个字符串 for(int i=0;i<s.size();i++) { if(s[i]>='0'&& s[i]<='9') sum++; } cout<<sum; return 0; }
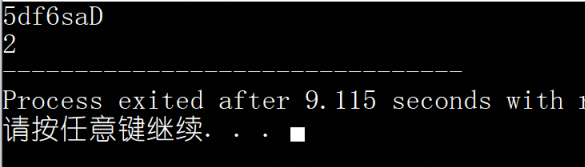
可以看出,跟字符数组的用法类似。
5.string类的一些用法
1.拼接字符串
比如我们想把“hello”和“world”拼在一起显示 “hello world”
方式1:通过 “+” 符号
int main() { string s1,s2,s3; s1="hello"; s2="world"; s3=s1+s2; cout<<s3; return 0; } //显示 helloworld
方式2:通过append方法
#include<iostream> #include<string> #include<cstdio> using namespace std; int main() { string s1,s2,s3; s1="hello"; s2="world"; s3=s1.append(s2); cout<<s3; return 0; } //显示helloworld
2.删除字符串 earse
erase参数一共有两个,表示要删除的起始位置和要删除的字符个数。
erase(start,num)
int main() { string s="123456789"; // s.erase(0,0); //结果为 123456789 // s.erase(0,1); //结果为 23456789 // s.erase(1,2); // 1456789 // s.erase(2,2); //1256789 s.erase(2,4); //12789 cout<<s; return 0; }
3. 字符串替换replace
replace的参数有三个,第一个是起始位置,第二个是要替换掉多少个,第三个是你要替换的字符串。
replace(start,num,str)
int main() { string s="123456789"; //s.replace(0,4,"w"); //结果w56789 s.replace(2,3,"what"); //结果是12what6789 cout<<s; return 0; }
4.查找字符串 find和rfind
首先,find和rfind的区别是,find是从前往后找,rfind是从后往前找。返回的是当前字符串第一次出现的位置。 如果没有找到,返回的是 s.npos。
#include<iostream> #include<string> #include<cstdio> using namespace std; int main() { string s="to be or not to be is a question?"; //查找一个字符串 cout<<s.find("to")<<endl; //结果是0; //倒叙查找一个字符串 cout<<s.rfind("to")<<endl; //结果是13 // 从某个位置开始查找一个字符串 cout<<s.find("to",3)<<endl;// 结果是13 //返回子串在母串中首次出现的位置 string s2="o"; cout<<s.find_first_of(s2)<<endl; //结果是1 //返回子串在母串中最后一次出现的位置 string s3="o"; cout<<s.find_last_of(s3)<<endl; // 结果是30 return 0; }
5.截取字符串substr
参数有两个 substr(start,length) 表示从什么地方开始,截取几个字符
#include<iostream> #include<string> #include<cstdio> using namespace std; int main() { string s="to be or not to be is a question?"; cout<<s.substr(0)<<endl; // 结果是 to be or not to be is a question? cout<<s.substr(0,2)<<endl; //结果是 to cout<<s.substr(1,4)<<endl; //结果是o be return 0; }
6.大小写转换
#include<iostream> #include<string> #include<cstdio> using namespace std; int main() { string s="to be or not to be is a question?"; for(int i=0;i<s.size();i++) s[i]=toupper(s[i]); //转大写 cout<<s; //输出 TO BE OR NOT TO BE IS A QUESTION? for(int i=0;i<s.size();i++) s[i]=tolower(s[i]); //转小写 cout<<s; //输出 to be or not to be is a question? return 0; }
7.字符串反转reverse
#include<iostream> #include<string> #include<cstdio> #include<algorithm> //使用reverse需要algorithm头文件 using namespace std; int main() { string s1="hello"; string s2="hello world"; reverse(s1.begin(),s1.end());// 反转s1 cout<<s1<<endl; //输出: olleh reverse(s2.begin(),s2.end()); //反转s2 cout<<s2<<endl; // 输出 dlrow olleh return 0; }
返回目录:C++