HTTP拦截器在开发过程中十分常见。在构建项目时,最好就直接创建一个HTTP拦截器,否则遇到以下几种问题,再进行改动就会十分浪费时间。
需要给所有的请求修改请求地址。
需要给所有的请求参数设置新的请求报文首部。
需要监听所有请求的状态码。
1.创建项目
前面的一些文章中简单说过创建angular项目的过程,可以简单参考。
项目创建完毕后,我们打开app.module.ts,我们导入httpClient。
app.module.ts:
import { HttpClientModule } from '@angular/common/http';
imports: [
HttpClientModule
],
然后我们创建HttpService.ts文件:
import { HttpClient } from "@angular/common/http"; import { Injectable } from "@angular/core"; import { from, Observable, of } from "rxjs"; import {catchError,map,tap,retry} from 'rxjs/operators' import { resolve } from "url"; @Injectable() export class HttpService{ private baseUrl='https://jsonplaceholder.typicode.com'; constructor( public http:HttpClient){} private extractData(res:Response){ const body=res; return body || {}; } private handleError<T>(operation ='opertion', result?:T){ return (error:any): Observable<T> => { console.error(error); console.log(`${operation} failed: ${error.message}`); return of(result as T); }; } }
baseUrl是网络请求通用的地址,这样发生网络请求时就不需要重复写了。extractData这个方法是用来对请求参数进行处理的,在这个例子中,我们虽然不需要处理请求参数,但是我们还是把这部分预留出来了。 handleError用来截获异常情况。这几个方法不需要对外公布,所以使用private进行修饰。
2.加入请求方法
import { HttpClient } from "@angular/common/http"; import { Injectable } from "@angular/core"; import { Observable, of } from "rxjs"; import {catchError,map,tap,retry} from 'rxjs/operators' @Injectable() export class HttpService{ private baseUrl='https://jsonplaceholder.typicode.com'; constructor( public http:HttpClient){} //get方法 public get(url:string):Observable<any>{ return this.http.get(this.baseUrl + url).pipe( retry(2), map(this.extractData), tap( ()=> {}), catchError(this.handleError('get',[])) ); } //post方法 public post(url:string, data:any={}):Observable<any>{ return this.http.post(this.baseUrl + url,data).pipe( retry(2), map(this.extractData), tap( ()=> {}), catchError(this.handleError('get',[])) ); } private extractData(res:Response){ const body=res; return body || {}; } private handleError<T>(operation ='opertion', result?:T){ return (error:any): Observable<T> => { console.error(error); console.log(`${operation} failed: ${error.message}`); return of(result as T); }; } }
我们在GET和POST请求中都是将baseUrl拼接在前面,retry是网络请求失败时重试的次数,map中存放了我们的请求参数,这个网络请求最终会返回一个可观察对象Observable。
然后在app.module.ts中加入 HttpService的引用
import { HttpService } from './http-service';
@NgModule({
...
providers: [HttpService ],
...
})
3.测试
我们在app.componet.ts文件中加入下面的代码:
constructor(private http:HttpService){
this.http.get('/todos/1').subscribe(res=>{
console.log(res)
})
}
然后就可以在控制台中看到下面返回的数据了
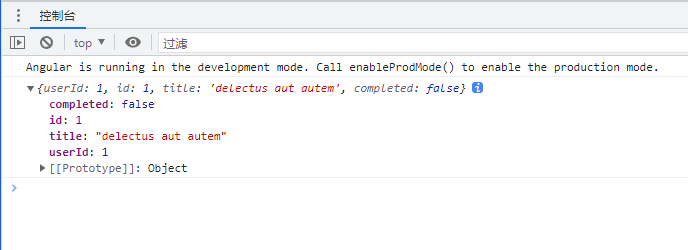