0.gateway简介
gateway是网关,是spring cloud 生态系统中的网关。gateway的主要作用是所有微服务的“入口”。
核心功能有三个:路由、权限控制、限流。
几个重要的概念:
- 路由:路由是网关最基础的部分,路由信息有一个ID、一个目的URL、一组断言和一组Filter组成。如果断言路由为真,则说明请求的URL和配置匹配
- 断言:匹配的规则。Java8中的断言函数。Spring Cloud Gateway中的断言函数输入类型是Spring5.0框架中的ServerWebExchange。Spring Cloud Gateway中的断言函数允许开发者去定义匹配来自于httprequest中的任何信息,比如请求头和参数等。
- 过滤器:一个标准的Spring webFilter。Spring cloud gateway中的filter分为两种类型的Filter,分别是Gateway Filter和Global Filter。过滤器Filter将会对请求和响应进行修改处理。
实现流程:
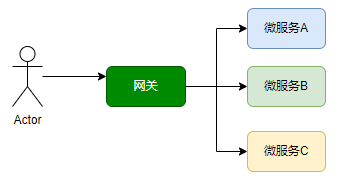
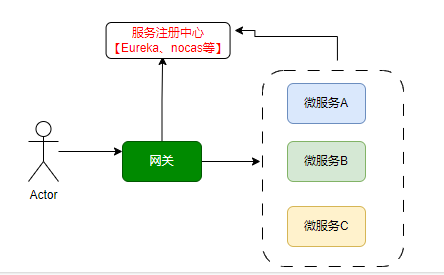
按照前面的框架,我们访问的框架是下面这样:
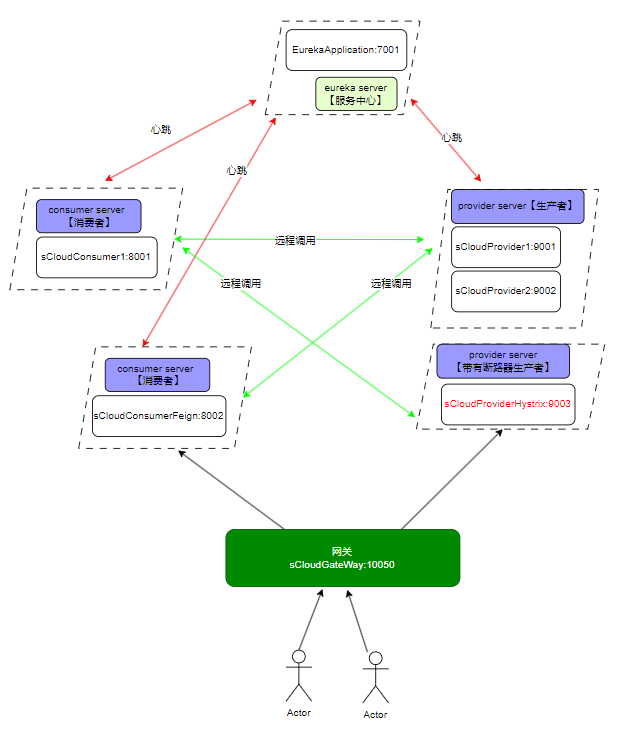
注意:网关只访问 微服务,不一定访问生产者还是消费者,在此举例一个消费者和一个生产者。
1.创建GateWay网关
我们右击父工程,创建一个模块,
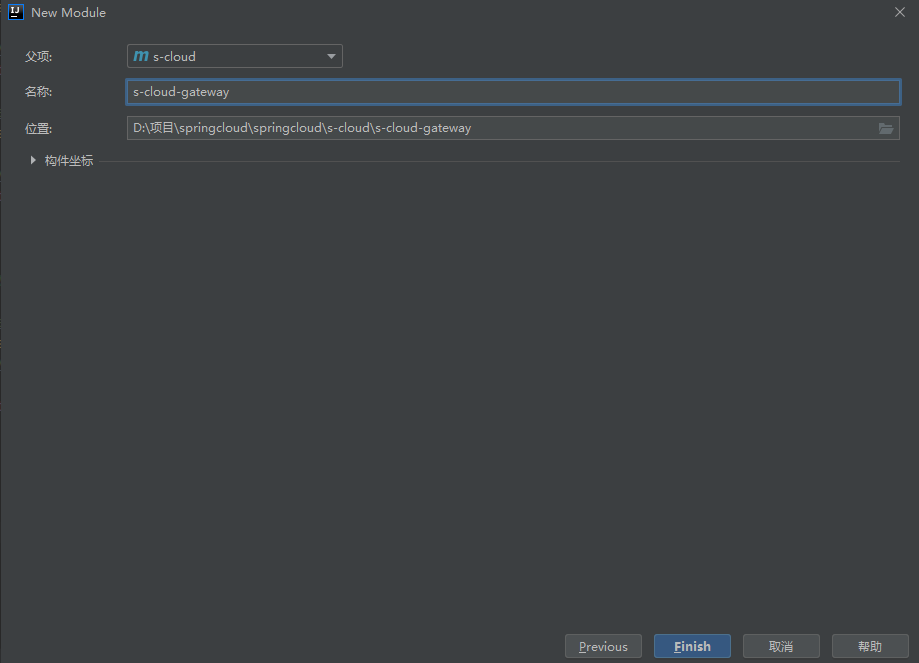
引入POM依赖文件
注意,这个地方不能引入spting boot的依赖,否则启动会报错。
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <parent> <artifactId>s-cloud</artifactId> <groupId>site.longkui</groupId> <version>0.0.1-SNAPSHOT</version> </parent> <modelVersion>4.0.0</modelVersion> <artifactId>s-cloud-gateway</artifactId> <dependencies> <!--eureka 客户端--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> <version>3.1.8-SNAPSHOT</version> </dependency> <!--gateway--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-gateway</artifactId> <version>3.1.8</version> <exclusions> <exclusion> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </exclusion> </exclusions> </dependency> </dependencies> </project>
配置文件 application.yml
server: port: 10050 #服务的端口 #spring相关配置 spring: application: name: service-gateway #服务的名称 #eureka配置 eureka: client: service-url: # 填写注册中心服务器地址 defaultZone: http://localhost:7001/eureka # 是否需要将自己注册到注册中心 register-with-eureka: true # 是否需要搜索服务信息 fetch-registry: true instance: # 使用ip地址注册到注册中心 prefer-ip-address: true # 注册中心列表中显示的状态参数 instance-id: ${spring.cloud.client.ip-address}:${server.port}
然后创建主启动类:
package site.longkui.scloudgateway; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.EnableEurekaClient; @SpringBootApplication @EnableEurekaClient public class sCloudGateWay { public static void main(String[] args) { SpringApplication.run(sCloudGateWay.class, args); } }
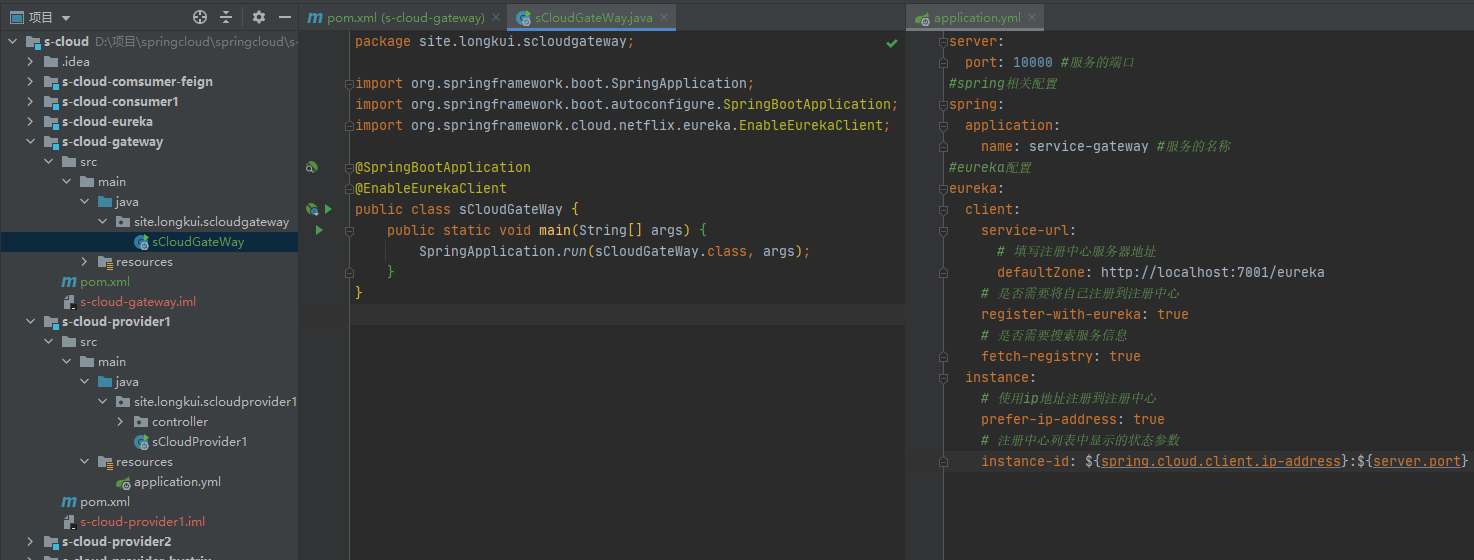
然后启动eureka和gateway,访问127.0.0.1:7001 可以看到网关正常注册。
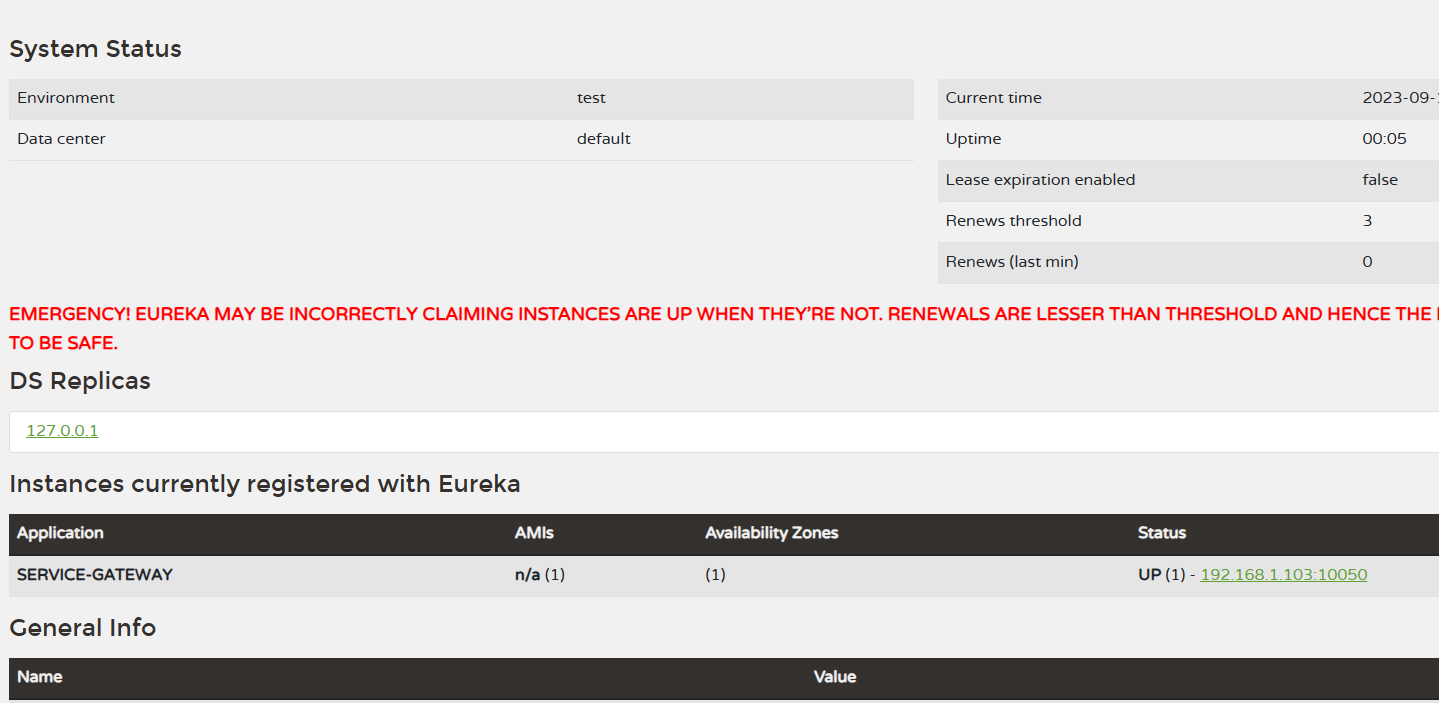
2.实现路由功能
我们需要修改gatway的application.yml文件,增加下面的代码(注意格式,强制要求空格)
server: port: 10050 #服务的端口 #spring相关配置 spring: application: name: service-gateway #服务的名称 #gateWay配置 cloud: gateway: routes: # 例子1 - id: s-cloud-gateway1 # 路由ID,命名随意,只要保证唯一性即可 uri: http://127.0.0.1:8002 # 设置一个地址,指向前面的一个消费者端口 predicates: - Method=GET,POST #只匹配GET和POST请求 #eureka配置 eureka: client: service-url: # 填写注册中心服务器地址 defaultZone: http://localhost:7001/eureka # 是否需要将自己注册到注册中心 register-with-eureka: true # 是否需要搜索服务信息 fetch-registry: true instance: # 使用ip地址注册到注册中心 prefer-ip-address: true # 注册中心列表中显示的状态参数 instance-id: ${spring.cloud.client.ip-address}:${server.port}
修改网关的配置文件,增加了两个路由,一个通过固定IP和端口的形式访问8002端口,另一个通过访问注册服务名的方式来访问,实际操作中一般采用后者。
predicates中配置了允许接口的访问规则,这里设置了GET和POST两种方式。
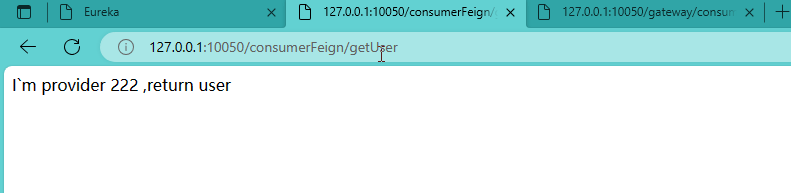
可以看出我们通过10050端口,访问了原来8002端口的功能,同样实现了原来的功能呢。
通过服务的方式进行访问:
server: port: 10050 #服务的端口 #spring相关配置 spring: application: name: service-gateway #服务的名称 #gateWay配置 cloud: gateway: routes: # 例子1 # - id: s-cloud-gateway1 # 路由ID,命名随意,只要保证唯一性即可 # uri: http://127.0.0.1:8002 # 设置一个地址,指向前面的一个消费者端口 # predicates: # - Method=GET,POST #只匹配GET和POST请求 #例子2 - id: s-cloud-gateway2 uri: lb://service-provider1 #以 lb:// 开头,后面的是注册在eureka上的服务名 predicates: - Method=GET,POST #只匹配GET和POST请求 #eureka配置 eureka: client: service-url: # 填写注册中心服务器地址 defaultZone: http://localhost:7001/eureka # 是否需要将自己注册到注册中心 register-with-eureka: true # 是否需要搜索服务信息 fetch-registry: true instance: # 使用ip地址注册到注册中心 prefer-ip-address: true # 注册中心列表中显示的状态参数 instance-id: ${spring.cloud.client.ip-address}:${server.port}

可以看出,实现了通过服务名的方式来访问微服务接口。