0.前言
接着上一篇继续讲。实际开发中,业务复杂时,访问的路由可能会比较复杂。比如你一个组件的功能下面还包含其他的子功能,那么你访问的路由可能是下面这个样子:
/city/shandong
/city/beijing
/city/henan
... ...
这个时候,可以看出,每个具体的城市都在 /city/目录下,也就是说,每个具体的城市都在city目录下,这个时候就要使用module来创建了。
2.创建带路由的Module
使用下面的的指令创建一个带路由的Module。
ng g m [Module名] --routing
ng g m city --routing
然后就可以看到带routing的Module了。
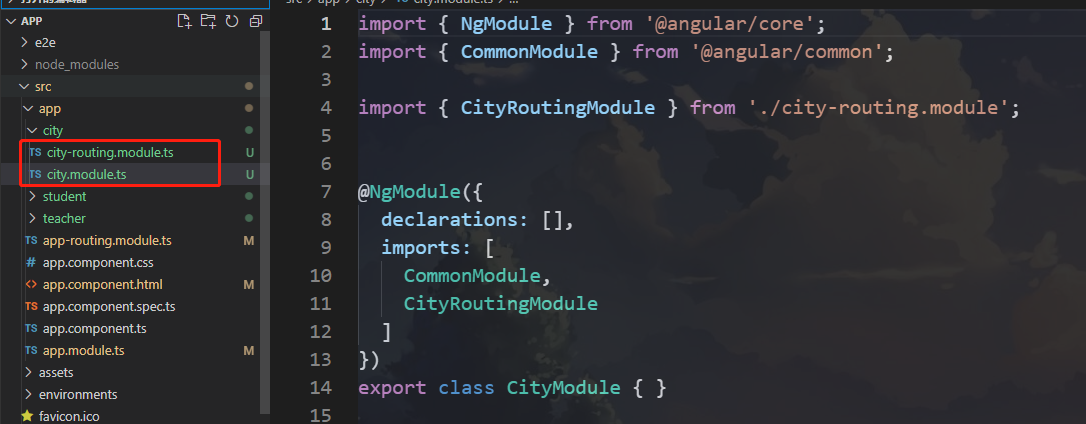
3.在moudle中创建组件
这一步中,我们需要在module中创建两个组件,首先cd 到我们刚才创建的city 文件夹目录下,然后执行下面的指令创建组件
ng g c shandong
和
ng g c beijing
再然后,我们在city-routing.module.ts中引入刚才创建的两个组件
import { NgModule } from '@angular/core'; import { Routes, RouterModule } from '@angular/router'; import { BeijingComponent } from './beijing/beijing.component'; import { ShandongComponent } from './shandong/shandong.component'; const routes: Routes = [ { // 山东 path:"shandong", component:ShandongComponent }, { //北京 path:"beijing", component:BeijingComponent } ]; @NgModule({ imports: [RouterModule.forChild(routes)], exports: [RouterModule] }) export class CityRoutingModule { }
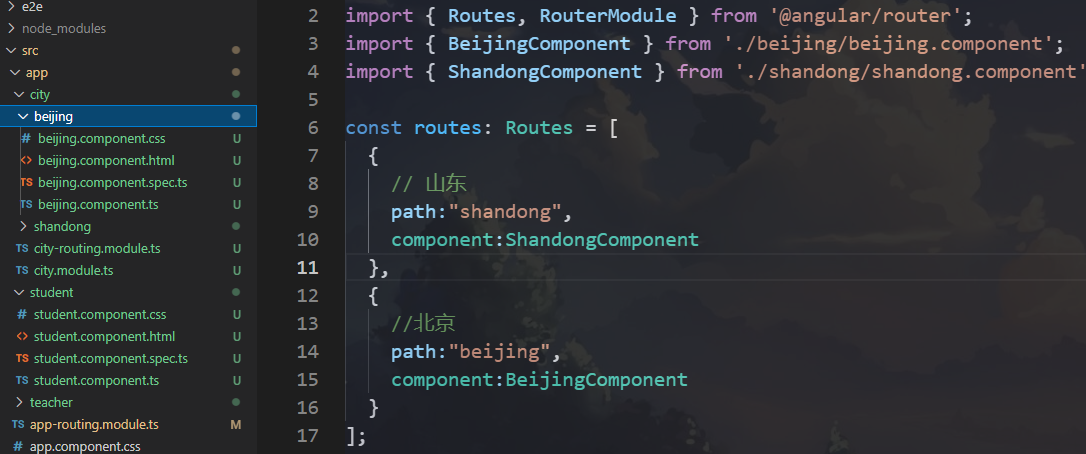
4.全局引入Module(懒加载)
我们把刚才新建的city这个Module。引入到全局的routing中(app-routing.module.ts),
import { NgModule } from '@angular/core'; import { Routes, RouterModule } from '@angular/router'; import { StudentComponent } from './student/student.component'; import { TeacherComponent } from './teacher/teacher.component'; const routes: Routes = [ {path:"tea",component:TeacherComponent}, {path:"stu",component:StudentComponent}, { path:"city", loadChildren: () => import('./city/city.module').then(m => m.CityModule), } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], // 初始化路由器,并让它开始监听浏览器的地址变化 exports: [RouterModule], }) export class AppRoutingModule { }
这个地方,我们通过 loadChildren(懒加载)引入创建的module。
然后通过/city/shandong 或者/city/beijing 来看到不同的页面内容。
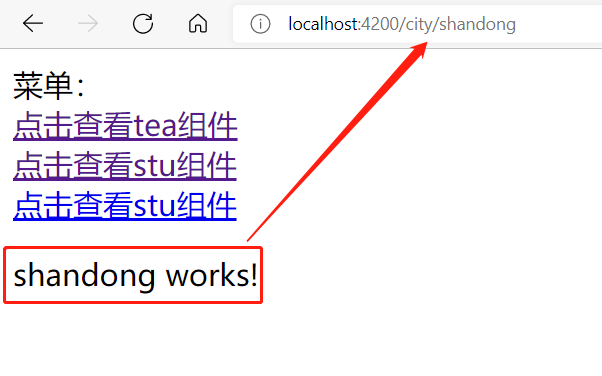
另外,还有下面这种写法:
loadChildren:"./city/city.module#CityModule",
3.重定义(redirectTo)
访问路由的时候,我们可以包装一下自己的路由,比如,你访问 /hh,直接跳转到 city/shandong
const routes: Routes = [ {path:"tea",component:TeacherComponent}, {path:"stu",component:StudentComponent}, { path:"city", loadChildren: () => import('./city/city.module').then(m => m.CityModule), //loadChildren:"./city/city.module#CityModule", }, { path:"hh", redirectTo:"/city/shandong" } ];