本文建议vscode先安装angular的插件,这样很多代码会自动生成

1.Constructor和OnInit的区别
当我们创建一个组件时,ts文件默认写成下面的样子
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-menu', templateUrl: './menu.component.html', styleUrls: ['./menu.component.scss'] }) export class MenuComponent implements OnInit { constructor() { } ngOnInit(): void { } }
(1)constructor是ES6引入类这个概念后出现的,和angular本身没有关系,constructor会在类生成实例时调用。angular无法控制constructor。举例来说,
class NewsComponent {
constructor(){
console.log("构造函数执行")
}
}
let news = new NewsComponent(); //当类被实例化时,构造函数被自动执行
constructor主要用来注入依赖。
(2)ngOnInit
ngOnInits是angular生命周期的一部分,ngOnInit
纯粹是通知开发者组件/指令已经被初始化完成了,此时组件/指令上的属性绑定操作以及输入操作已经完成,也就是说在ngOnInit
函数中我们已经能够操作组件/指令中被传入的数据了.
生命周期介绍
名称 | 作用 |
ngOnChanges() | 当绑定的数值发生变化时触发 |
ngOnInit() | 在第一轮ngOnChanges()完成之后调用,只调用一次 |
ngOnDestory() | 在Angular销毁指令或组件之前立即调用 |
ngDoCheck() | 紧跟在每次执行变更检测时的 ngOnChanges() 和 首次执行变更检测时的 ngOnInit() 后调用 |
ngAfterViewInit() | 第一次 ngAfterContentChecked() 之后调用,只调用一次 |
ngAfterViewChecked() | ngAfterViewInit() 和每次 ngAfterContentChecked() 之后调用。 |
ngAfterContentInit() | 第一次 ngDoCheck() 之后调用,只调用一次。 |
ngAfterContentChecked() | ngAfterContentInit() 和每次 ngDoCheck() 之后调用 |
其中:constructor、ngOnInit()、ngAfterContentInit()、ngAfterViewInit() 、ngOnDestroy只调用一次。其余组件在相应动作时进行触发。
我们可以把这些函数都添加上。
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-menu', templateUrl: './menu.component.html', styleUrls: ['./menu.component.scss'] }) export class MenuComponent implements OnInit { constructor() { } ngOnInit(): void { } ngOnChanges(changes: SimpleChanges): void { //Called before any other lifecycle hook. Use it to inject dependencies, but avoid any serious work here. //Add '${implements OnChanges}' to the class. } ngDoCheck(): void { //Called every time that the input properties of a component or a directive are checked. Use it to extend change detection by performing a custom check. //Add 'implements DoCheck' to the class. } ngAfterContentChecked(): void { //Called after every check of the component's or directive's content. //Add 'implements AfterContentChecked' to the class. } ngAfterContentInit(): void { //Called after ngOnInit when the component's or directive's content has been initialized. //Add 'implements AfterContentInit' to the class. } ngAfterViewInit(): void { //Called after ngAfterContentInit when the component's view has been initialized. Applies to components only. //Add 'implements AfterViewInit' to the class. } ngAfterViewChecked(): void { //Called after every check of the component's view. Applies to components only. //Add 'implements AfterViewChecked' to the class. } ngOnDestroy(): void { //Called once, before the instance is destroyed. //Add 'implements OnDestroy' to the class. } }
2.自定义函数
除了上面angular有的函数,我们还可以自定义函数。
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-menu', templateUrl: './menu.component.html', styleUrls: ['./menu.component.scss'] }) export class MenuComponent implements OnInit { constructor() { } ngOnInit(): void { } //自定义函数 hello(){ console.log("自定义函数hello执行") } }
然后,我们在html文件中调用即可
<button (click)="hello()">这是menu里的button</button>
然后,我们就可以在控制台看到消息了。
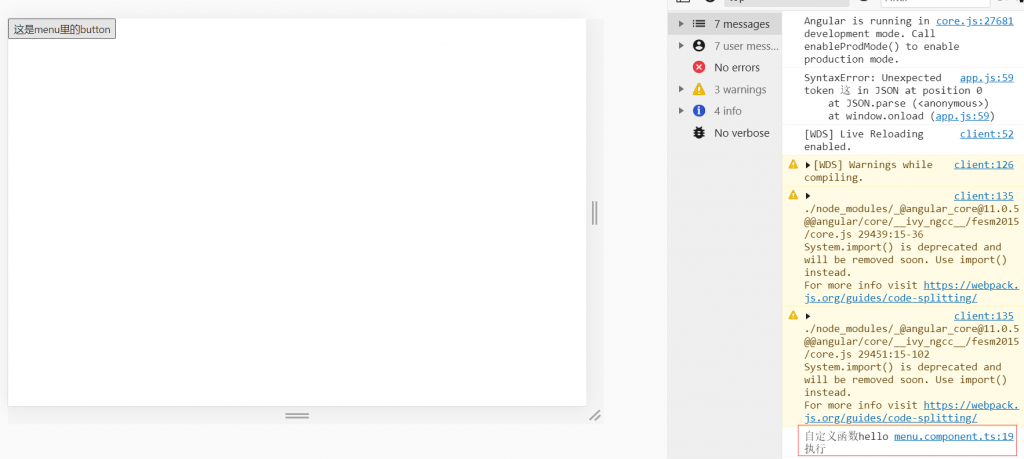
返回目录:前端